1 2 3 4
| import torch import d2lzh_pytorch import os import pandas as pd
|
1 2 3 4 5 6
| x=torch.arange(12) print(x) x.shape x.numel x.reshape(3,4) y=torch.zeros(2,3,4)
|
tensor([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
1 2 3 4 5 6 7 8 9 10 11 12 13
| x = torch.tensor([[1, 2, 3], [3, 4, 5], [4, 3, 2]]) print(x) x = torch.tensor([2.0, 2, 2, 2]) print(x) x = torch.arange(12, dtype=torch.float32).reshape((3, 4)) print(x) y = torch.tensor([[2.0, 1, 5, 3], [3, 2, 2, 3], [3, 2, 3, 3]]) print(y) torch.cat((x, y), dim=0), torch.cat((x, y), dim=1)
x==y
x.sum()
|
tensor([[1, 2, 3],
[3, 4, 5],
[4, 3, 2]])
tensor([2., 2., 2., 2.])
tensor([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.]])
tensor([[2., 1., 5., 3.],
[3., 2., 2., 3.],
[3., 2., 3., 3.]])
tensor(66.)
1 2 3 4 5
| a = torch.arange(3).reshape((3, 1)) b = torch.arange(2).reshape((1, 2)) a, b a+b
|
tensor([[0, 1],
[1, 2],
[2, 3]])
1 2 3 4 5
| print(x) x[-1], x[1:3]
|
tensor([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.]])
(tensor([ 8., 9., 10., 11.]),
tensor([[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.]]))
1 2 3 4 5 6 7 8 9
| x=torch.zeros(3,4) print(x) x[1,2]=9 print(x) x[0:2,:]=12 print(x) before = id(y) y+=x id(y)==before
|
tensor([[0., 0., 0., 0.],
[0., 0., 0., 0.],
[0., 0., 0., 0.]])
tensor([[0., 0., 0., 0.],
[0., 0., 9., 0.],
[0., 0., 0., 0.]])
tensor([[12., 12., 12., 12.],
[12., 12., 12., 12.],
[ 0., 0., 0., 0.]])
True
1 2 3
| before=id(x) x+=y id(x)==before
|
True
1 2 3 4 5 6 7 8 9 10 11
| os.makedirs(os.path.join('./', "data"), exist_ok=True) data_file = os.path.join('./', 'data', 'house_tiny.csv') with open(data_file, 'w') as f: f.write('NumRoom,Alley,Price\n') f.write('Na,Pave,127500\n') f.write('2,Na,199999\n') f.write('5,Jager,199999\n') f.write('3,Bruse,199999\n')
data = pd.read_csv(data_file) data
|
|
NumRoom |
Alley |
Price |
0 |
Na |
Pave |
127500 |
1 |
2 |
Na |
199999 |
2 |
5 |
Jager |
199999 |
3 |
3 |
Bruse |
199999 |
1 2 3 4 5 6
| a = torch.arange(12) b = a.reshape((3, 4)) b[:] = 2 print(a) print(b)
|
tensor([2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2])
tensor([[2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2]])
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| x = torch.tensor([3.0]) y = torch.tensor([3.9]) x+y
x = torch.arange(4) print(len(x)) print(x.shape)
a = torch.arange(20).reshape(5, 4) print(a) print(a.T)
a = torch.arange(20, dtype=torch.float32).reshape(5, 4) b = a.clone() print(b)
print(a*b) a = 2
|
4
torch.Size([4])
tensor([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11],
[12, 13, 14, 15],
[16, 17, 18, 19]])
tensor([[ 0, 4, 8, 12, 16],
[ 1, 5, 9, 13, 17],
[ 2, 6, 10, 14, 18],
[ 3, 7, 11, 15, 19]])
tensor([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.],
[12., 13., 14., 15.],
[16., 17., 18., 19.]])
tensor([[ 0., 1., 4., 9.],
[ 16., 25., 36., 49.],
[ 64., 81., 100., 121.],
[144., 169., 196., 225.],
[256., 289., 324., 361.]])
1 2 3 4 5 6 7
| a = torch.arange(12).reshape(3, 4) print(a) sum_1 = a.sum(axis=0) print(sum_1) sum_2 = a.sum(axis=1) print(sum_2)
|
tensor([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11]])
tensor([12, 15, 18, 21])
tensor([ 6, 22, 38])
1 2 3
| a = torch.arange(12, dtype=float).reshape(3, 4) print(a.mean()) print(a.sum()/a.numel())
|
tensor(5.5000, dtype=torch.float64)
tensor(5.5000, dtype=torch.float64)
1 2 3 4 5 6 7
| a = torch.arange(12, dtype=float).reshape(3, 4) sum_a = a.sum(axis=1, keepdim=True) print(sum_a) sum1=a.cumsum(axis=0) print(sum1)
|
tensor([[ 6.],
[22.],
[38.]], dtype=torch.float64)
tensor([[ 0., 1., 2., 3.],
[ 4., 6., 8., 10.],
[12., 15., 18., 21.]], dtype=torch.float64)
1 2 3 4 5 6 7 8
| a = torch.arange(12, dtype=float) b = torch.ones(12, dtype=float) print(torch.dot(a, b))
a = torch.arange(12, dtype=float).reshape(3, 4) b = torch.ones((3, 4)) torch.sum(a*b)
|
tensor(66., dtype=torch.float64)
tensor(66., dtype=torch.float64)
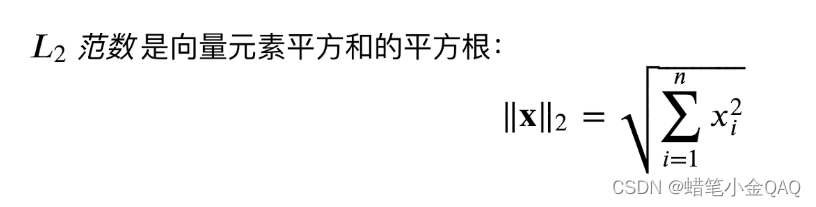
1 2
| u = torch.tensor([3.0, 4, 5]) torch.norm(u)
|
tensor(7.0711)
1 2 3 4 5 6
| a = torch.ones((2, 5, 4)) print(a) print(a.sum(axis=1).shape) print(a.sum(axis=0).shape) print(a.sum(axis=[0, 2]).shape)
|
tensor([[[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.]],
[[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.]]])
torch.Size([2, 4])
torch.Size([5, 4])
torch.Size([5])
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| a = torch.arange(4, dtype=float, requires_grad=True) print(a.grad)
b = 2*torch.dot(a, a) b.backward() print(a.grad)
a.grad.zero_() y=a.sum() y.backward() print(a.grad)
a.grad.zero_() y=a*a y.sum().backward() a.grad
a.grad.zero_() y=a*a u=y.detach() z=u*a z.sum().backward() a.grad==u
|
None
tensor([ 0., 4., 8., 12.], dtype=torch.float64)
tensor([1., 1., 1., 1.], dtype=torch.float64)
tensor([True, True, True, True])
1 2 3 4 5 6 7 8 9 10
| a = torch.arange(4, dtype=float).reshape(2, 2) b = torch.arange(4, dtype=float).reshape(2, 2) result = torch.empty(2, 2) print(result) torch.add(a, b, out=result) print(result) print(a) print(b) a.add_(b) print(a)
|
tensor([[0., 2.],
[0., 0.]])
tensor([[0., 2.],
[4., 6.]])
tensor([[0., 1.],
[2., 3.]], dtype=torch.float64)
tensor([[0., 1.],
[2., 3.]], dtype=torch.float64)
tensor([[0., 2.],
[4., 6.]], dtype=torch.float64)
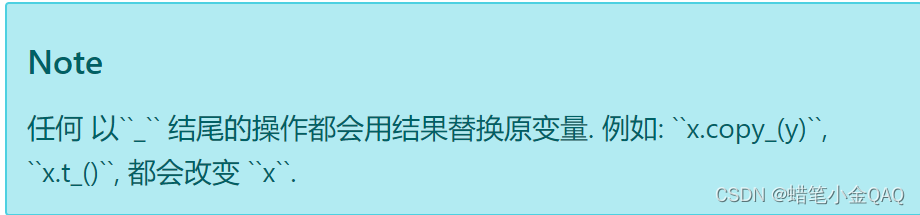
1 2 3 4
| x = torch.arange(12, dtype=float).reshape(3, 4) print(x) print(x[:, 2]) print(x[1:3, :])
|
tensor([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.]], dtype=torch.float64)
tensor([ 2., 6., 10.], dtype=torch.float64)
tensor([[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.]], dtype=torch.float64)